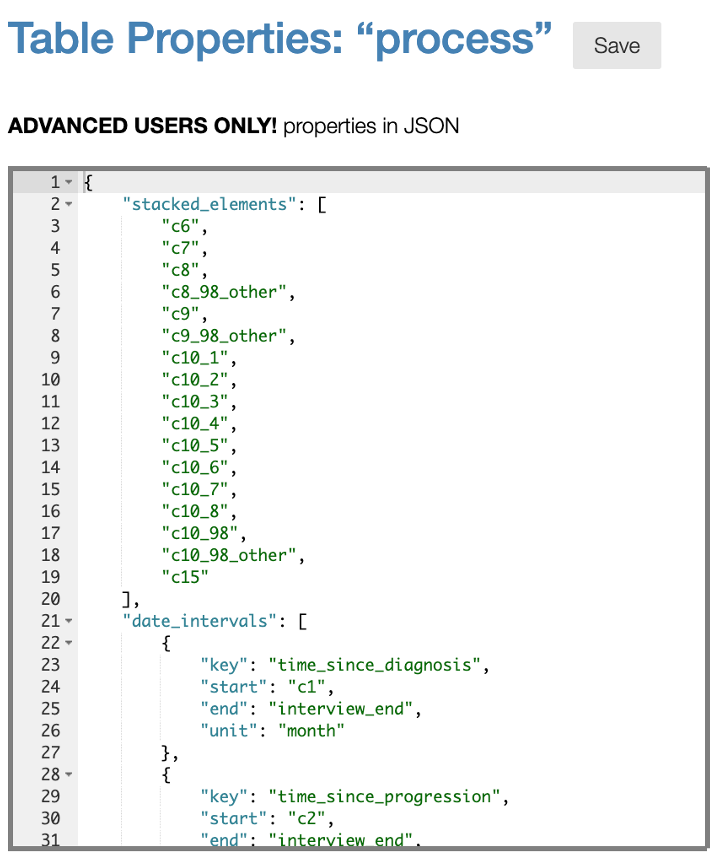
Table properties lets you store variable definitions separately from the process logic. A variable is just a “named storage” for data, and many people like to define all the variables they plan to use at the top of a data process so that they are easy to find and modify.
With table properties, your variable definitions are on a separate page which keeps your process logic cleaner and makes reading and editing code easier. This is especially useful when variable definitions are expansive which can take up a lot of rows of your data process and make it hard to locate specific code.
Values accepted in table properties
Tables properties is defined as an object. Objects in Javascript are a collection of properties, and each property is a a key (name) and value pair.
Properties can have the following values:
- String
- Numeric
- Boolean
- An array
- An object
Practical use
We recommend variables with long definitions like a list of questions or complex functions be stored in table properties.
Variables you can store in table properties:
- Questions that you want to operate on in the data process
- Data columns you want to merge with your survey data from an auxiliary data file
- Data columns you want to stack (i.e. a patient chart module)
- Data columns you want to delete
- Date objects with information for time interval calculations
- Objects with group name (key) and children definitions
- Functions (reusable blocks of code that do a specific task)
How to get to table properties
Each data process has its own table properties. You can get to table properties from the process setup page by pressing the “Properties” button.
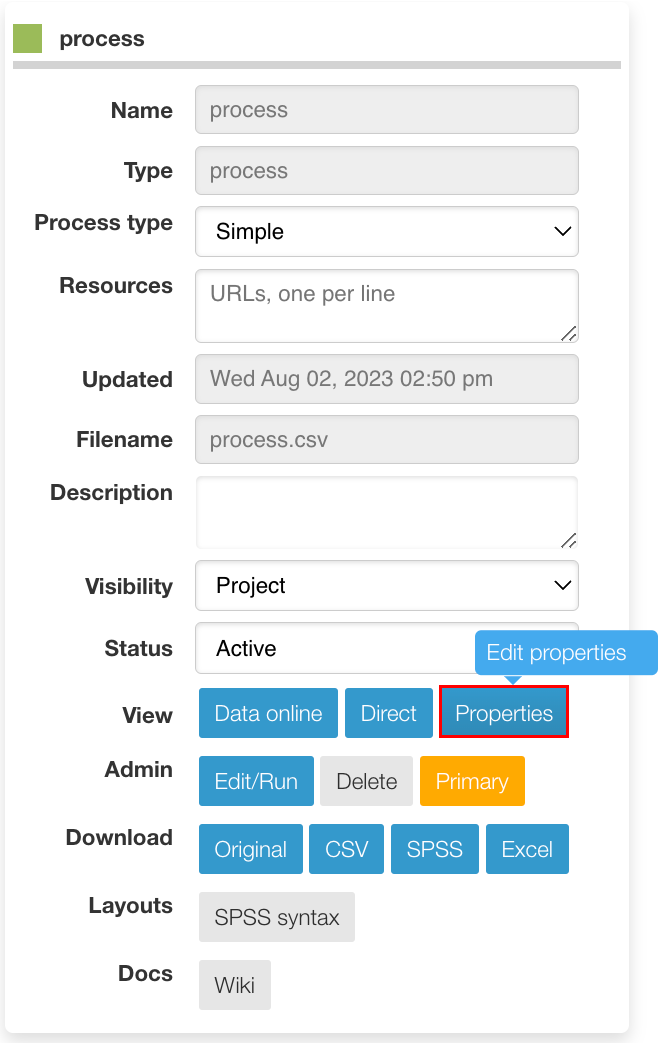
You can also get to table properties from the code view page by pressing “Properties…” next to the “Run…” button.
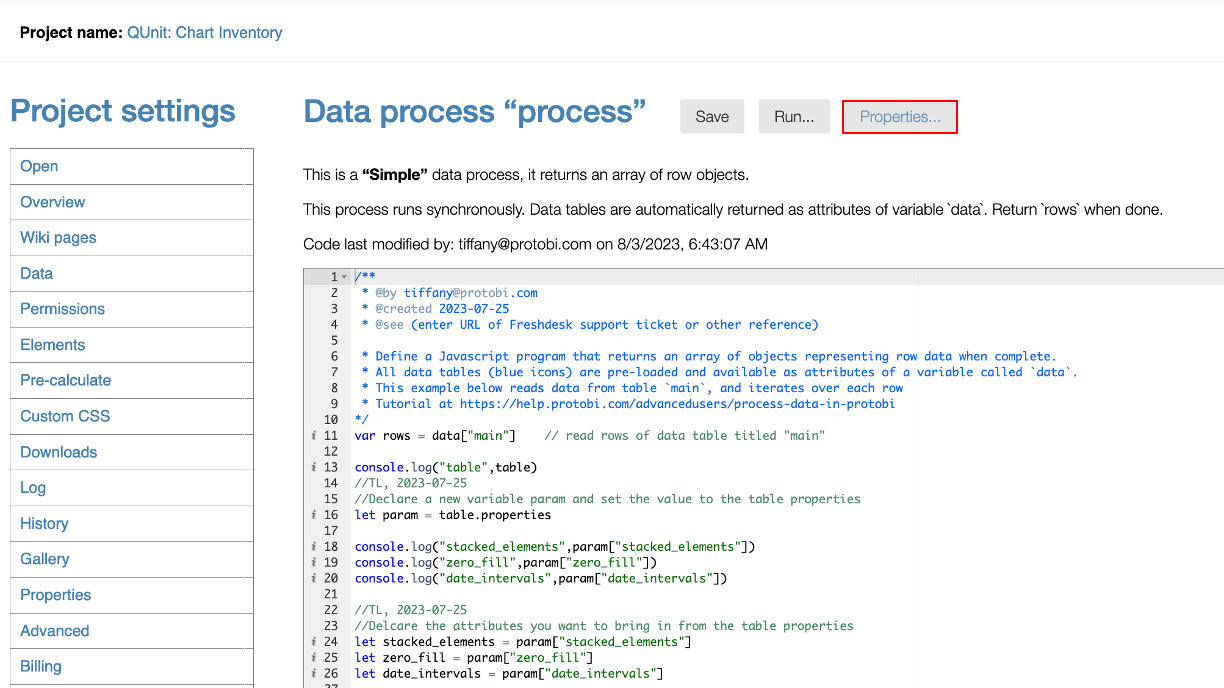
Table properties page
In the table properties example below, we include a few common examples of pre-defined variables that can make the data process long and hard to manage. We recommend you store these definitions in table properties.
Store keys and keep arrays for left_join data merges
The “keys" and “keep” properties are arrays and we reference these variables in code that joins additional data to the survey data file.
Store keys and groups to reference in zero_fill code
The zero_fill property is an array of objects with keys and children. The keys and children are used in the zero-fill code to replace missing values with zeros in question batteries that sum to 100.
Expand to see example code for table properties.
Table properties
{
"keys": [
"respid"
],
"keep": [
"q6_coded",
"q15_coded",
"q23_coded",
"q24_coded_1",
"q25_coded_2",
"q26_coded_3",
"q27_coded_4",
"q28_coded_5",
"q29_coded_6"
],
"zero_fill": [
{
"key": "q20",
"children": [
"q20_1",
"q20_2",
"q20_3",
"q20_4",
"q20_5",
"q20_6",
"q20_7",
"q20_8",
"q20_98"
]
},
{
"key": "q25",
"children": [
"q25_1",
"q25_2",
"q25_3",
"q25_4",
"q25_5",
"q25_6",
"q25_7",
"q25_8",
"q25_98"
]
},
{
"key": "q30",
"children": [
"q30_1",
"q30_2",
"q30_3",
"q30_4",
"q30_5",
"q30_6",
"q30_7",
"q30_8",
"q30_98"
]
}
]
}
Data process page
Expand the data process code below to see how the table properties defined above are used.
Data process
/**
* @by tiffany@protobi.com
* @created 2023-07-25
* @see (enter URL of Freshdesk support ticket or other reference)
* Define a Javascript program that returns an array of objects representing row data when complete.
* All data tables (blue icons) are pre-loaded and available as attributes of a variable called `data`.
* This example below reads data from table `main`, and iterates over each row
* Tutorial at https://help.protobi.com/advancedusers/process-data-in-protobi
*/
var rows = data["main"] // read rows of data table titled "main"
var OE = data["OE"] //auxiliary data file
//TL, 2023-07-25
//Declare a new variable param and set the value to the table properties
let param = table.properties
//Declare the attributes you want to bring in from the table properties
let keys = param["keys"]
let keep = param["keep"]
let zero_fill = param["zero_fill"]
//TL, 2023-07-25
//Join in coded open-end data
//Keys are merge keys.
//Keeps are all the fields to add to the main data file or replace existing data.
rows = Protobi.left_join(
rows, //left table (project's existing data)
OE, //right table (data to be joined into project)
keys, //join keys
keep //the variables from the right table to join
);
rows.forEach(function(row) {
//TL, 2023/07/25, zero-fill code
//Zero and [NA] fill question batteries
zero_fill.forEach(function(entry) {
key = entry.key;
children = entry.children;
children.forEach(function(child) {
row[key] = (row[key] || 0) + (+row[child] || 0);
})
if (row[key] == 0) {
row[key] = "";
children.forEach(function(child) {
row[child] = ""; // NA fill those respondents that has 0 total
})
}
else {
children.forEach(function(child) {
row[child] = (row[child] || 0);
})
}
})
})
return rows;